How to automatically open Rhino and run a Grasshopper file using a batch file.
A batch file is a file that allows Windows to run a list of commands automatically. They are simple text files with file extension .bat that you can easily write yourself. This post is a good tutorial on how to make a batch file.
Batch files were used in the Pollination project in Grasshopper to automate Grasshopper tasks between multiple computers. Andrew Heumann explained here how to write the batch file to start Rhino and run a Grasshopper file.
What to write in the Grasshopper batch file
Copy this into a text document, and change the fields to your own file locations. Save the file, and change the extension from .txt to .bat.
@ECHO OFF
cd **PATH TO DIRECTORY CONTAINING GH FILE**
"**PATH TO RHINO.EXE**" /nosplash /runscript="-grasshopper editor load document open **GRASSHOPPER FILE NAME** _enter" "**PATH TO ASSOCIATED RHINO FILE**"
For example, I have a Rhino and a GH file on my desktop, respectively called random.gh and random2.3dm.
@ECHO OFF
cd C:\Users\jrams\Desktop
"C:\Program Files\Rhinoceros 5 (64-bit)\System\rhino.exe" /nosplash /runscript="-grasshopper editor load document open C:\Users\jrams\Desktop\random.gh _enter" "C:\Users\jrams\Desktop\random2.3dm"
Now you can just double-click on the batch file you have made. Rhino should now open with your Grasshopper file.
Seeing an error with the characters ╗┐?
The ECHO OFF command should stop the command prompt from being visible. Sometimes, you might get an error like this. This error stops the first line in your batch file from being executed properly, in this case disabling the ECHO OFF command.
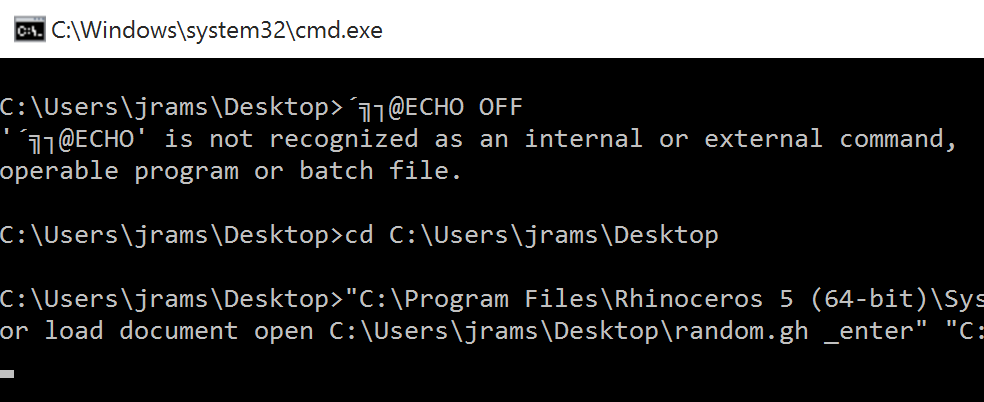
Long story short, your text file’s encoding is incompatible with the command prompt. To fix, in Notepad, save with ANSI encoding. Or in Sublime, go to File then Save With Encoding. Choose UTF-8 (NOT UTF-8 with BOM).